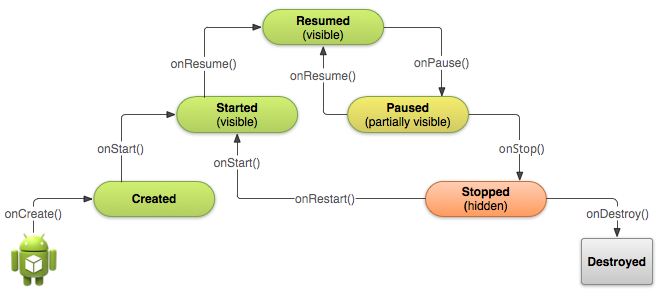
Figure 1. A simplified illustration of the Activity lifecycle, expressed as a step pyramid. This shows how, for every callback used to take the activity a step toward the Resumed state at the top, there’s a callback method that takes the activity a step down. The activity can also return to the resumed state from the Paused and Stopped state.
用户在Home界面选择你的app图标时系统将调用你指定的”launcher”或是”main” activity的onCreate方法。该activity在AndroidManifest.xml中被指定,它必须有一个包含MAIN action和LAUNCHER category的,缺少其中任何一个你的app图标就不会再Home界面显示。
系统调用onDestroy一般是在按顺序调用onPause和onStop之后,但有一个例外:当在onCreate中调用finish方法时将会触发系统直接调用onDestroy。
* Does not crash if the user receives a phone call or switches to another app while using your app.
* Does not consume valuable system resources when the user is not actively using it.
* Does not lose the user’s progress if they leave your app and return to it at a later time.
* Does not crash or lose the user’s progress when the screen rotates between landscape and portrait orientation.
当activity处于部分可见(如activity上弹出对话框时)时系统将调用onPause方法。onPause中需要处理一些必要的数据保存工作,但不要处理很耗时的工作(如写数据库)。很耗时的工作将影响activity状态的转换(转到onStop),应该放在onStop中处理。
有些正常的app行为会导致activity被destroy,如:用户按了返回键、activity自己调用了finish方法。另一些情况,如activity处于stop状态很长一段时间了,或者当前拥有焦点的activity需要更多系统资源必须终止后台进程时,或者旋转屏幕时,也会导致activity被destroy。但对后一种情况系统通过Bundle保存了当时activity的信息。
系统会在发生后一种情况时调用onSaveInstanceState来保存activity当时的信息,因此我们可以通过重写该方法实现保存自定义数据。当系统重新创建该activity时,我们可以通过onCreate和onRestoreInstanceState来恢复。当有保存的数据时,系统会在onStart之后调用onRestoreInstaceState;而当用onCreate来恢复时需要注意判断参数是否为空,因为新建而非重建的activity也会调用onCreate。